Scalars#
Python defines only one type of a particular data class (there is only one integer type, one floating-point type, etc.). This can be convenient in applications that don’t need to be concerned with all the ways data can be represented in a computer. For scientific computing, however, more control is often needed.
In NumPy, there are 24 new fundamental Python types to describe different types of scalars. These type descriptors are mostly based on the types available in the C language that CPython is written in, with several additional types compatible with Python’s types.
Array scalars have the same attributes and methods as ndarrays
. [1] This allows one to treat items of an array partly on
the same footing as arrays, smoothing out rough edges that result when
mixing scalar and array operations.
Array scalars live in a hierarchy (see the Figure below) of data
types. They can be detected using the hierarchy: For example,
isinstance(val, np.generic)
will return True
if val is
an array scalar object. Alternatively, what kind of array scalar is
present can be determined using other members of the data type
hierarchy. Thus, for example isinstance(val, np.complexfloating)
will return True
if val is a complex valued type, while
isinstance(val, np.flexible)
will return true if val is one
of the flexible itemsize array types (str_
,
bytes_
, void
).
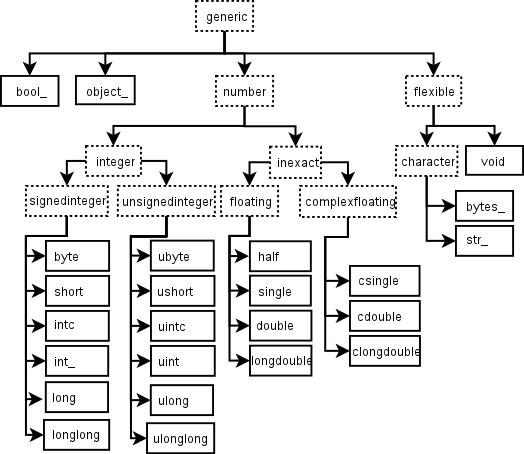
Figure: Hierarchy of type objects representing the array data
types. Not shown are the two integer types intp
and
uintp
which are used for indexing (the same as the
default integer since NumPy 2).#
Built-in scalar types#
The built-in scalar types are shown below. The C-like names are associated with character codes, which are shown in their descriptions. Use of the character codes, however, is discouraged.
Some of the scalar types are essentially equivalent to fundamental Python types and therefore inherit from them as well as from the generic array scalar type:
Array scalar type |
Related Python type |
Inherits? |
---|---|---|
Python 2 only |
||
yes |
||
yes |
||
yes |
||
yes |
||
no |
||
no |
||
no |
The bool_
data type is very similar to the Python
bool
but does not inherit from it because Python’s
bool
does not allow itself to be inherited from, and
on the C-level the size of the actual bool data is not the same as a
Python Boolean scalar.
Warning
The int_
type does not inherit from the
int
built-in under Python 3, because type int
is no
longer a fixed-width integer type.
Tip
The default data type in NumPy is double
.
- class numpy.generic[source]#
Base class for numpy scalar types.
Class from which most (all?) numpy scalar types are derived. For consistency, exposes the same API as
ndarray
, despite many consequent attributes being either “get-only,” or completely irrelevant. This is the class from which it is strongly suggested users should derive custom scalar types.
Integer types#
Note
The numpy integer types mirror the behavior of C integers, and can therefore be subject to Overflow errors.
Signed integer types#
- class numpy.byte[source]#
Signed integer type, compatible with C
char
.- Character code:
'b'
- Alias on this platform (Darwin arm64):
numpy.int8
: 8-bit signed integer (-128
to127
).
- class numpy.short[source]#
Signed integer type, compatible with C
short
.- Character code:
'h'
- Alias on this platform (Darwin arm64):
numpy.int16
: 16-bit signed integer (-32_768
to32_767
).
- class numpy.intc[source]#
Signed integer type, compatible with C
int
.- Character code:
'i'
- Alias on this platform (Darwin arm64):
numpy.int32
: 32-bit signed integer (-2_147_483_648
to2_147_483_647
).
- class numpy.int_[source]#
Default signed integer type, 64bit on 64bit systems and 32bit on 32bit systems.
- Character code:
'l'
- Alias on this platform (Darwin arm64):
numpy.int64
: 64-bit signed integer (-9_223_372_036_854_775_808
to9_223_372_036_854_775_807
).- Alias on this platform (Darwin arm64):
numpy.intp
: Signed integer large enough to fit pointer, compatible with Cintptr_t
.
Unsigned integer types#
- class numpy.ubyte[source]#
Unsigned integer type, compatible with C
unsigned char
.- Character code:
'B'
- Alias on this platform (Darwin arm64):
numpy.uint8
: 8-bit unsigned integer (0
to255
).
- class numpy.ushort[source]#
Unsigned integer type, compatible with C
unsigned short
.- Character code:
'H'
- Alias on this platform (Darwin arm64):
numpy.uint16
: 16-bit unsigned integer (0
to65_535
).
- class numpy.uintc[source]#
Unsigned integer type, compatible with C
unsigned int
.- Character code:
'I'
- Alias on this platform (Darwin arm64):
numpy.uint32
: 32-bit unsigned integer (0
to4_294_967_295
).
- class numpy.uint[source]#
Unsigned signed integer type, 64bit on 64bit systems and 32bit on 32bit systems.
- Character code:
'L'
- Alias on this platform (Darwin arm64):
numpy.uint64
: 64-bit unsigned integer (0
to18_446_744_073_709_551_615
).- Alias on this platform (Darwin arm64):
numpy.uintp
: Unsigned integer large enough to fit pointer, compatible with Cuintptr_t
.
Inexact types#
- class numpy.inexact[source]#
Abstract base class of all numeric scalar types with a (potentially) inexact representation of the values in its range, such as floating-point numbers.
Note
Inexact scalars are printed using the fewest decimal digits needed to
distinguish their value from other values of the same datatype,
by judicious rounding. See the unique
parameter of
format_float_positional
and format_float_scientific
.
This means that variables with equal binary values but whose datatypes are of different precisions may display differently:
>>> f16 = np.float16("0.1")
>>> f32 = np.float32(f16)
>>> f64 = np.float64(f32)
>>> f16 == f32 == f64
True
>>> f16, f32, f64
(0.1, 0.099975586, 0.0999755859375)
Note that none of these floats hold the exact value \(\frac{1}{10}\);
f16
prints as 0.1
because it is as close to that value as possible,
whereas the other types do not as they have more precision and therefore have
closer values.
Conversely, floating-point scalars of different precisions which approximate the same decimal value may compare unequal despite printing identically:
>>> f16 = np.float16("0.1")
>>> f32 = np.float32("0.1")
>>> f64 = np.float64("0.1")
>>> f16 == f32 == f64
False
>>> f16, f32, f64
(0.1, 0.1, 0.1)
Floating-point types#
- class numpy.half[source]#
Half-precision floating-point number type.
- Character code:
'e'
- Alias on this platform (Darwin arm64):
numpy.float16
: 16-bit-precision floating-point number type: sign bit, 5 bits exponent, 10 bits mantissa.
- class numpy.single[source]#
Single-precision floating-point number type, compatible with C
float
.- Character code:
'f'
- Alias on this platform (Darwin arm64):
numpy.float32
: 32-bit-precision floating-point number type: sign bit, 8 bits exponent, 23 bits mantissa.
- class numpy.double(x=0, /)[source]#
Double-precision floating-point number type, compatible with Python
float
and Cdouble
.- Character code:
'd'
- Alias on this platform (Darwin arm64):
numpy.float64
: 64-bit precision floating-point number type: sign bit, 11 bits exponent, 52 bits mantissa.
Complex floating-point types#
- class numpy.complexfloating[source]#
Abstract base class of all complex number scalar types that are made up of floating-point numbers.
- class numpy.csingle[source]#
Complex number type composed of two single-precision floating-point numbers.
- Character code:
'F'
- Alias on this platform (Darwin arm64):
numpy.complex64
: Complex number type composed of 2 32-bit-precision floating-point numbers.
- class numpy.cdouble(real=0, imag=0)[source]#
Complex number type composed of two double-precision floating-point numbers, compatible with Python
complex
.- Character code:
'D'
- Alias on this platform (Darwin arm64):
numpy.complex128
: Complex number type composed of 2 64-bit-precision floating-point numbers.
Other types#
- class numpy.bool[source]#
Boolean type (True or False), stored as a byte.
Warning
The
bool
type is not a subclass of theint_
type (thebool
is not even a number type). This is different than Python’s default implementation ofbool
as a sub-class ofint
.- Character code:
'?'
- class numpy.datetime64[source]#
If created from a 64-bit integer, it represents an offset from
1970-01-01T00:00:00
. If created from string, the string can be in ISO 8601 date or datetime format.When parsing a string to create a datetime object, if the string contains a trailing timezone (A ‘Z’ or a timezone offset), the timezone will be dropped and a User Warning is given.
Datetime64 objects should be considered to be UTC and therefore have an offset of +0000.
>>> np.datetime64(10, 'Y') numpy.datetime64('1980') >>> np.datetime64('1980', 'Y') numpy.datetime64('1980') >>> np.datetime64(10, 'D') numpy.datetime64('1970-01-11')
See Datetimes and timedeltas for more information.
- Character code:
'M'
- class numpy.timedelta64[source]#
A timedelta stored as a 64-bit integer.
See Datetimes and timedeltas for more information.
- Character code:
'm'
Note
The data actually stored in object arrays
(i.e., arrays having dtype object_
) are references to
Python objects, not the objects themselves. Hence, object arrays
behave more like usual Python lists
, in the sense
that their contents need not be of the same Python type.
The object type is also special because an array containing
object_
items does not return an object_
object
on item access, but instead returns the actual object that
the array item refers to.
The following data types are flexible: they have no predefined
size and the data they describe can be of different length in different
arrays. (In the character codes #
is an integer denoting how many
elements the data type consists of.)
- class numpy.flexible[source]#
Abstract base class of all scalar types without predefined length. The actual size of these types depends on the specific
numpy.dtype
instantiation.
- class numpy.bytes_[source]#
A byte string.
When used in arrays, this type strips trailing null bytes.
- Character code:
'S'
- class numpy.str_[source]#
A unicode string.
This type strips trailing null codepoints.
>>> s = np.str_("abc\x00") >>> s 'abc'
Unlike the builtin
str
, this supports the Buffer Protocol, exposing its contents as UCS4:>>> m = memoryview(np.str_("abc")) >>> m.format '3w' >>> m.tobytes() b'a\x00\x00\x00b\x00\x00\x00c\x00\x00\x00'
- Character code:
'U'
- class numpy.void(length_or_data, /, dtype=None)[source]#
Create a new structured or unstructured void scalar.
- Parameters:
- length_or_dataint, array-like, bytes-like, object
One of multiple meanings (see notes). The length or bytes data of an unstructured void. Or alternatively, the data to be stored in the new scalar when
dtype
is provided. This can be an array-like, in which case an array may be returned.- dtypedtype, optional
If provided the dtype of the new scalar. This dtype must be “void” dtype (i.e. a structured or unstructured void, see also Structured datatypes).
New in version 1.24.
Notes
For historical reasons and because void scalars can represent both arbitrary byte data and structured dtypes, the void constructor has three calling conventions:
np.void(5)
creates adtype="V5"
scalar filled with five\0
bytes. The 5 can be a Python or NumPy integer.np.void(b"bytes-like")
creates a void scalar from the byte string. The dtype itemsize will match the byte string length, here"V10"
.When a
dtype=
is passed the call is roughly the same as an array creation. However, a void scalar rather than array is returned.
Please see the examples which show all three different conventions.
Examples
>>> np.void(5) np.void(b'\x00\x00\x00\x00\x00') >>> np.void(b'abcd') np.void(b'\x61\x62\x63\x64') >>> np.void((3.2, b'eggs'), dtype="d,S5") np.void((3.2, b'eggs'), dtype=[('f0', '<f8'), ('f1', 'S5')]) >>> np.void(3, dtype=[('x', np.int8), ('y', np.int8)]) np.void((3, 3), dtype=[('x', 'i1'), ('y', 'i1')])
- Character code:
'V'
Warning
See Note on string types.
Numeric Compatibility: If you used old typecode characters in your
Numeric code (which was never recommended), you will need to change
some of them to the new characters. In particular, the needed
changes are c -> S1
, b -> B
, 1 -> b
, s -> h
, w ->
H
, and u -> I
. These changes make the type character
convention more consistent with other Python modules such as the
struct
module.
Sized aliases#
Along with their (mostly)
C-derived names, the integer, float, and complex data-types are also
available using a bit-width convention so that an array of the right
size can always be ensured. Two aliases (numpy.intp
and numpy.uintp
)
pointing to the integer type that is sufficiently large to hold a C pointer
are also provided.
- numpy.int8[source]#
- numpy.int16#
- numpy.int32#
- numpy.int64#
Aliases for the signed integer types (one of
numpy.byte
,numpy.short
,numpy.intc
,numpy.int_
,numpy.long
andnumpy.longlong
) with the specified number of bits.Compatible with the C99
int8_t
,int16_t
,int32_t
, andint64_t
, respectively.
- numpy.uint8[source]#
- numpy.uint16#
- numpy.uint32#
- numpy.uint64#
Alias for the unsigned integer types (one of
numpy.ubyte
,numpy.ushort
,numpy.uintc
,numpy.uint
,numpy.ulong
andnumpy.ulonglong
) with the specified number of bits.Compatible with the C99
uint8_t
,uint16_t
,uint32_t
, anduint64_t
, respectively.
- numpy.intp[source]#
Alias for the signed integer type (one of
numpy.byte
,numpy.short
,numpy.intc
,numpy.int_
,numpy.long
andnumpy.longlong
) that is used as a default integer and for indexing.Compatible with the C
Py_ssize_t
.- Character code:
'n'
Changed in version 2.0: Before NumPy 2, this had the same size as a pointer. In practice this is almost always identical, but the character code
'p'
maps to the Cintptr_t
. The character code'n'
was added in NumPy 2.0.
- numpy.uintp[source]#
Alias for the unsigned integer type that is the same size as
intp
.Compatible with the C
size_t
.- Character code:
'N'
Changed in version 2.0: Before NumPy 2, this had the same size as a pointer. In practice this is almost always identical, but the character code
'P'
maps to the Cuintptr_t
. The character code'N'
was added in NumPy 2.0.
- numpy.float96#
- numpy.float128#
Alias for
numpy.longdouble
, named after its size in bits. The existence of these aliases depends on the platform.
- numpy.complex192#
- numpy.complex256#
Alias for
numpy.clongdouble
, named after its size in bits. The existence of these aliases depends on the platform.
Attributes#
The array scalar objects have an array priority
of NPY_SCALAR_PRIORITY
(-1,000,000.0). They also do not (yet) have a ctypes
attribute. Otherwise, they share the same attributes as arrays:
The integer value of flags. |
|
Tuple of array dimensions. |
|
Tuple of bytes steps in each dimension. |
|
The number of array dimensions. |
|
Pointer to start of data. |
|
The number of elements in the gentype. |
|
The length of one element in bytes. |
|
Scalar attribute identical to the corresponding array attribute. |
|
Get array data-descriptor. |
|
The real part of the scalar. |
|
The imaginary part of the scalar. |
|
A 1-D view of the scalar. |
|
Scalar attribute identical to the corresponding array attribute. |
|
Array protocol: Python side |
|
Array protocol: struct |
|
Array priority. |
|
sc.__array_wrap__(obj) return scalar from array |
Indexing#
See also
Array scalars can be indexed like 0-dimensional arrays: if x is an array scalar,
x[()]
returns a copy of array scalarx[...]
returns a 0-dimensionalndarray
x['field-name']
returns the array scalar in the field field-name. (x can have fields, for example, when it corresponds to a structured data type.)
Methods#
Array scalars have exactly the same methods as arrays. The default behavior of these methods is to internally convert the scalar to an equivalent 0-dimensional array and to call the corresponding array method. In addition, math operations on array scalars are defined so that the same hardware flags are set and used to interpret the results as for ufunc, so that the error state used for ufuncs also carries over to the math on array scalars.
The exceptions to the above rules are given below:
sc.__array__(dtype) return 0-dim array from scalar with specified dtype |
|
sc.__array_wrap__(obj) return scalar from array |
|
Scalar method identical to the corresponding array attribute. |
|
Scalar method identical to the corresponding array attribute. |
|
Helper for pickle. |
|
Scalar method identical to the corresponding array attribute. |
Utility method for typing:
|
Return a parametrized wrapper around the |
Defining new types#
There are two ways to effectively define a new array scalar type
(apart from composing structured types dtypes from
the built-in scalar types): One way is to simply subclass the
ndarray
and overwrite the methods of interest. This will work to
a degree, but internally certain behaviors are fixed by the data type of
the array. To fully customize the data type of an array you need to
define a new data-type, and register it with NumPy. Such new types can only
be defined in C, using the NumPy C-API.