Mentorship¶
fname = "data/2020/numpy_survey_results.tsv"
column_names = [
'participated', 'role', 'mentor_paid', 'mentor_motivation',
'mentor_motivation_other', 'mentor_connect', 'mentor_connect_other',
'mentor_activities', 'mentor_activities_other', 'mentee_charged',
'mentee_connect', 'mentee_connect_other', 'mentee_activities',
'mentee_activities_other', 'satisfaction', 'interested'
]
mentorship_dtype = np.dtype({
"names": column_names,
"formats": ['<U1024'] * len(column_names),
})
data = np.loadtxt(
fname, delimiter='\t', skiprows=3, dtype=mentorship_dtype,
usecols=range(56, 74), comments=None
)
We asked survey participants about their experiences with mentorship programs related to OSS scientific software. Of the 1236 survey respondents, 122 (10%) reported participating in some form of mentorship program dealing with scientific software: 67 (55%) as mentors, 18 (15%) as mentees, and 37 (30%) in both capacities1.
participant_mask = data['participated'] == 'Yes'
glue(
'num_mentorship_participants',
gluval(participant_mask.sum(), data.shape[0]),
display=False
)
mentor_mask, mentee_mask, both_mask = (
data['role'] == key for key in ('Mentor', 'Mentee', 'Both')
)
num_mentors = mentor_mask.sum()
num_mentees = mentee_mask.sum()
num_both = both_mask.sum()
glue(
'num_mentors',
gluval(num_mentors, participant_mask.sum()),
display=False
)
glue('num_mentees', gluval(num_mentees, participant_mask.sum()), display=False)
glue('num_both', gluval(num_both, participant_mask.sum()), display=False)
Paid vs. Unpaid Programs¶
14 (13%) of respondents who served as mentors reported being paid by the program, and 8 (15%) mentees reported being charged fees.
num_paid_mentors = np.sum(data['mentor_paid'] == 'Yes')
num_charged_mentees = np.sum(data['mentee_charged'] == 'Yes')
glue(
'mentors_paid',
gluval(num_paid_mentors, (num_mentors + num_both)),
display=False,
)
glue(
'mentees_charged',
gluval(num_charged_mentees, (num_mentees + num_both)),
display=False,
)
Mentor Motivations¶
We asked mentors to share their motivations for serving as OSS mentors.
all_mentors_mask = mentor_mask | both_mask
motivations = data['mentor_motivation'][all_mentors_mask]
motivations = motivations[motivations != '']
num_resp = motivations.shape[0]
motivations = flatten(motivations)
labels, cnts = np.unique(motivations, return_counts=True)
I = np.argsort(cnts)
labels, cnts = labels[I], cnts[I]
cnts = 100 * cnts / num_resp
fig, ax = plt.subplots(figsize=(12, 8))
ax.barh(np.arange(len(labels)), cnts)
ax.set_yticks(np.arange(len(labels)))
ax.set_yticklabels(labels)
ax.set_xlabel('Percentage of Mentors')
fig.tight_layout()
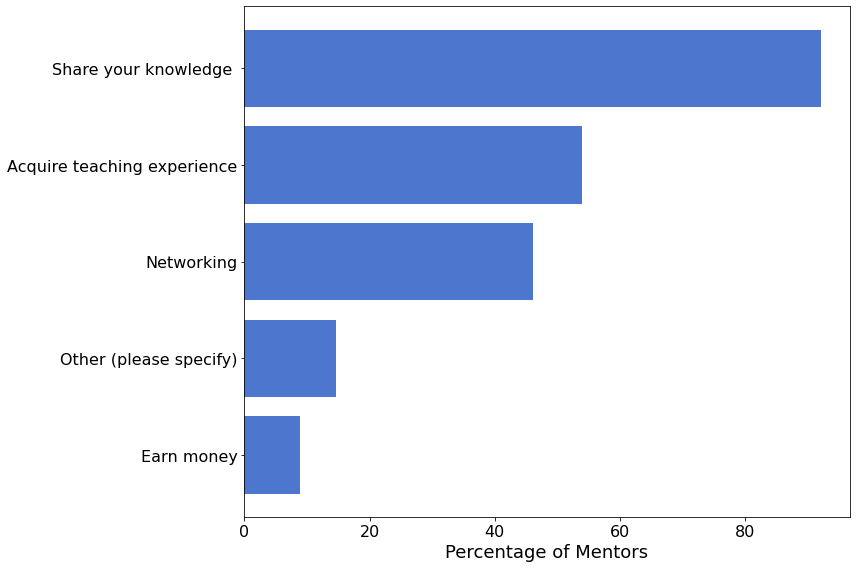
Mentor Connections¶
We asked both mentors and mentees how they were matched with their counterparts.
fig, ax = plt.subplots(figsize=(12, 8))
for start_ind, (key, label) in enumerate(zip(
('mentor_connect', 'mentee_connect'),
('Mentors', 'Mentees')
)):
cnxn_data = data[key][data[key] != '']
num_resp = cnxn_data.shape[0]
labels, cnts = np.unique(flatten(cnxn_data), return_counts=True)
# Plot
ax.barh(
np.arange(start_ind, 2 * len(labels), 2),
100 * cnts / num_resp,
align='edge',
label=label,
)
ax.set_yticks(np.arange(start_ind, 2 * len(labels), 2))
ax.set_yticklabels(labels)
ax.set_xlabel('Percentage of Participants')
ax.legend()
fig.tight_layout()
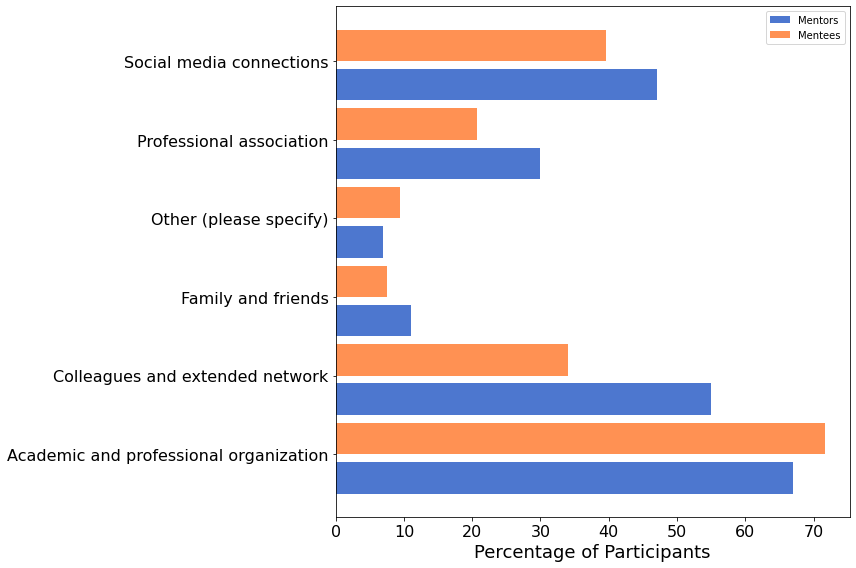
Mentorship Activities¶
We asked participants what sorts of activities they engaged in as part of the mentorship program.
# NOTE: not every activity was reported by both mentors/mentees
labels = np.unique(flatten(data['mentor_activities']))[3:]
fig, ax = plt.subplots(figsize=(12, 8))
for start_ind, (key, label) in enumerate(zip(
('mentor_activities', 'mentee_activities'),
('Mentors', 'Mentees')
)):
activities_data = data[key][data[key] != '']
num_resp = activities_data.shape[0]
activities_data = np.array(flatten(activities_data))
cnts = np.array([np.sum(activities_data == act) for act in labels])
# Plot
ax.barh(
np.arange(start_ind, 2 * len(labels), 2),
100 * cnts / num_resp,
align='edge',
label=label,
)
# Manual modification to one category name
labels[labels == 'Attend a lecture'] = 'Attend an Event'
ax.set_yticks(np.arange(start_ind, 2 * len(labels), 2))
ax.set_yticklabels(labels)
ax.set_xlabel('Percentage of Participants')
ax.legend()
fig.tight_layout()
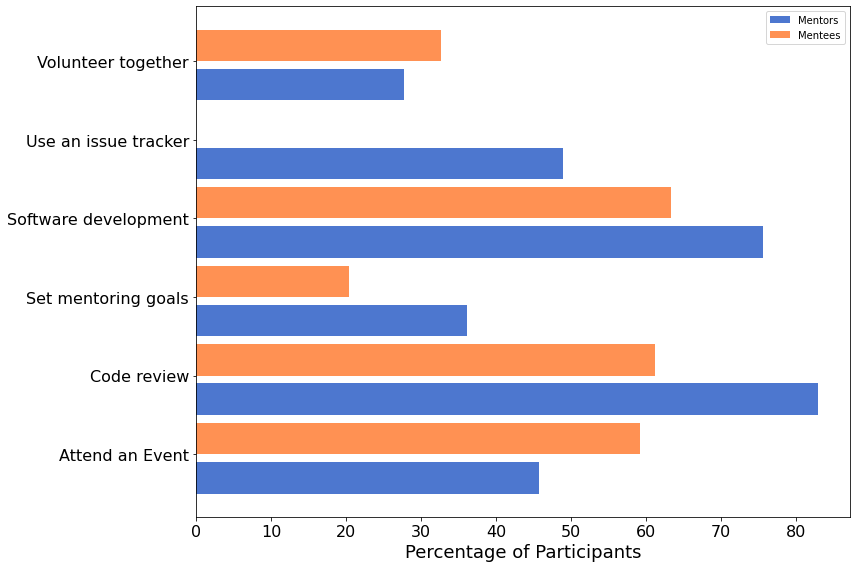
Program Satisfaction¶
We asked mentees how satisfied they were with their experience in the mentorship program(s).
satisfaction = data['satisfaction'][data['satisfaction'] != '']
labels, cnts = np.unique(satisfaction, return_counts=True)
fig, ax = plt.subplots(figsize=(8, 8))
ax.pie(cnts, labels=labels, autopct='%1.1f%%')
fig.tight_layout()
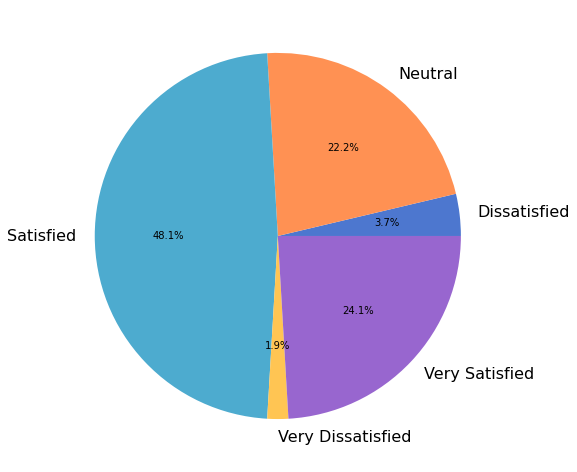
Interest in a NumPy Mentorship Program¶
Finally, we asked survey participants whether they would be interested in a formal NumPy mentorship program. Of the 978 participants who responded to this inquiry, 592 (61%) said that they would be interested, including 73 (70%) of mentors and 44 (80%) of mentees who have previously participated in other OSS mentorship programs.
all_mentees_mask = mentee_mask | both_mask
num_resp = np.sum(data['interested'] != '')
num_yes = np.sum(data['interested'] == 'Yes')
num_former_mentors_yes = np.sum(data['interested'][all_mentors_mask] == 'Yes')
num_former_mentees_yes = np.sum(data['interested'][all_mentees_mask] == 'Yes')
glue('num_responded_mentorship_interest', num_resp, display=False)
glue('interested_in_mentorship', gluval(num_yes, num_resp), display=False)
glue(
'former_mentors',
gluval(num_former_mentors_yes, all_mentors_mask.sum()),
display=False
)
glue(
'former_mentees',
gluval(num_former_mentees_yes, all_mentees_mask.sum()),
display=False
)
- 1
No distinction is made for those who have participated in multiple different mentorship programs.